New C++ Job: Software Developer C++ (m/f/x) - IKARUS anti.virus in Vienna #cppjobs
https://www.meetingcpp.com/jobs/items/Software-Developer-Cpp--m-f-x----IKARUS-anti-virus.html
#cpp
#cplusplus
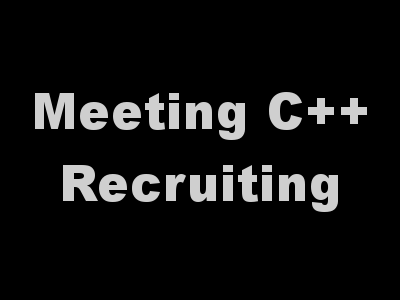
New C++ Job: Software Developer C++ (m/f/x) - IKARUS anti.virus in Vienna #cppjobs
https://www.meetingcpp.com/jobs/items/Software-Developer-Cpp--m-f-x----IKARUS-anti-virus.html
#cpp
#cplusplus
Looking for Employers for the Job Fair and the Jobs Newsletter
https://www.meetingcpp.com/meetingcpp/news/items/Looking-for-Employers-for-the-Job-Fair-and-the-Jobs-Newsletter.html
#cpp
#cplusplus
C++Now 2025 SESSION ANNOUNCEMENT: Making Your Program Faster - On Multihreading and Automatic Compiler Vectorization by Ivica Bogosavljevic
https://schedule.cppnow.org/session/2025/making-your-program-faster/
Register now at https://cppnow.org/registration/
Xcode 16.3 contains a big apple-clang update
https://developer.apple.com/documentation/xcode-release-notes/xcode-16_3-release-notes
Discussions: https://discu.eu/q/https://developer.apple.com/documentation/xcode-release-notes/xcode-16_3-release-notes
Just finished working on a C++ #cuesheet parser. It uses #boost #spirit and so far it seems to work OK. It's a bit rough around the edges but usable. I haven't released it yet but happy to attach a GPL3+ licence and upload it if anyone would like to give it a try #cpp #libre #programming #coding #gpl
Rewrote the existing C code for a simple molecular dynamics simulation in Rust.
What a brilliant language. So clean and clear.
Fun experience. Took me 3 days. (No LLM help haha).
#CppPollOfToday: In which industry fields have you worked as a C++ dev?
https://meetingcpp.com/mcpp/survey/?q=81
#cplusplus
#cpp
Also, this is a huge argument for a societal shift back in favour of defined benefit pensions, and doubling the #cpp.
Should you use final?
https://www.sandordargo.com/blog/2025/04/09/no-final-mock
Discussions: https://discu.eu/q/https://www.sandordargo.com/blog/2025/04/09/no-final-mock
Book of the Day: C++ Initialization Story by Bartlomiej Filipek
https://meetingcpp.com/mcpp/books/book.php?hash=031796a1aeb9e05c2b88b23599e711fe7e98bc8a
#cplusplus
#cpp
Hey engineers!
Any leads for #OpenSource #CPP #software that uses C++ StdPar parallelism as their (main) parallelization scheme?
Would be quite interested in learning about such apps and domains where that is used.
What’s New in vcpkg (March 2025)
https://devblogs.microsoft.com/cppblog/whats-new-in-vcpkg-march-2025/
#cpp
#cplusplus
Given
float max = numeric_limits<float>::max();
float x = max * max - max * max;
What is the value of x?
Submit your job openings to the Meeting C++ jobs newsletter!
https://meetingcpp.com/mcpp/recruiting/jobsubmission.php
#cpp
#cplusplus
Should you use final?
https://www.sandordargo.com/blog/2025/04/09/no-final-mock
#cpp
#cplusplus
This YT short summarizes changes needed to have a Qt Quick app work with both Qt 5 and Qt 6. It primarily focuses on required changes to #QML and #CMake files, but it also mentions other aspects of porting C++ and shaders: https://www.youtube.com/shorts/zQ5hNgwuC4g #QtDev #Cpp
https://www.youtube.com/shorts/zQ5hNgwuC4g
Everything you need to know about installing and reusing CMake projects
https://www.youtube.com/watch?v=OMx3cZj_hoo
#cpp
#cplusplus